The Util global
The Util
global type provides common utility methods. Below are the methods provided
by the Util
type:
Util.Math
Util.Html
Util.Table
Util.Url
Util.Image
Util.Math
This is a simple utility method for composing a LatexOutput
type. This is intended to be used
in conjunction with the Display
function.
1:
|
Util.Math("f(x) = g(x) / 10") |> Display |
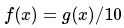
Util.Html
This is another utility method used for composing an HtmlOutput
type. This is intended to be used
in conjuction with the Display
function.
1:
|
Util.Html(@"<div style=""background-color: pink; font-weight: bold;"">Would you like some toast?</div>") |> Display |
Would you like some toast?
Util.Table
Converts a sequence of objects into a TableOutput
type. This is intended to be used in conjunction with
the Display
function.
1: 2: 3: 4: 5: 6: 7: 8: 9: 10: 11: 12: |
type MyType = { FirstName: string; LastName: string } let records = [| { FirstName = "Duane"; LastName = "Dibbley" } { FirstName = "Arnold"; LastName = "Rimmer" } { FirstName = "Dave"; LastName = "Lister" } { FirstName = ""; LastName = "Kryten" } { FirstName = ""; LastName = "Holly" } { FirstName = "Kristine"; LastName = "Kochanski" } |] Util.Table(records) |> Display |
First Name | Last Name |
---|---|
Duane | Dibbley |
Arnold | Rimmer |
David | Lister |
Dave | Lister |
Kryten | |
Holly | |
Kristine | Kochanski |
Can also be used to only contain certain columns.
1:
|
Util.Table(records, [| "LastName" |]) |> Display |
LastName |
---|
Dibbley |
Rimmer |
Lister |
Kryten |
Holly |
Kochanski |
Util.Url
Downloads a URL and converts it for display using the content-type of the url for display.
This is intended to be used in conjunction with the Display
function.
1:
|
Util.Url("https://www.google.com/images/srpr/logo11w.png") |> Display |
Util.Image
Loads an image from either a byte array.
1: 2: |
let bytes = System.IO.File.ReadAllBytes("img/toaster.png") Util.Image(bytes, "image/png") |> Display |
Or loads an image from a file name
1:
|
Util.Image("img/toaster.png") |> Display |
type Util = Util
Full name: IfSharp.Kernel.Globals.Globals.Util
Full name: IfSharp.Kernel.Globals.Globals.Util
static member Util.Math : str:string -> LatexOutput
val Display : ('a -> unit)
Full name: IfSharp.Kernel.Globals.Globals.Display
Full name: IfSharp.Kernel.Globals.Globals.Display
static member Util.Html : str:string -> HtmlOutput
type MyType =
{FirstName: string;
LastName: string;}
Full name: Utils.MyType
{FirstName: string;
LastName: string;}
Full name: Utils.MyType
MyType.FirstName: string
Multiple items
val string : value:'T -> string
Full name: Microsoft.FSharp.Core.Operators.string
--------------------
type string = System.String
Full name: Microsoft.FSharp.Core.string
val string : value:'T -> string
Full name: Microsoft.FSharp.Core.Operators.string
--------------------
type string = System.String
Full name: Microsoft.FSharp.Core.string
MyType.LastName: string
val records : MyType []
Full name: Utils.records
Full name: Utils.records
static member Util.Table : items:seq<'A> * ?propertyNames:seq<string> -> TableOutput
static member Util.Url : url:string -> BinaryOutput
val bytes : byte []
Full name: Utils.bytes
Full name: Utils.bytes
namespace System
namespace System.IO
type File =
static member AppendAllLines : path:string * contents:IEnumerable<string> -> unit + 1 overload
static member AppendAllText : path:string * contents:string -> unit + 1 overload
static member AppendText : path:string -> StreamWriter
static member Copy : sourceFileName:string * destFileName:string -> unit + 1 overload
static member Create : path:string -> FileStream + 3 overloads
static member CreateText : path:string -> StreamWriter
static member Decrypt : path:string -> unit
static member Delete : path:string -> unit
static member Encrypt : path:string -> unit
static member Exists : path:string -> bool
...
Full name: System.IO.File
static member AppendAllLines : path:string * contents:IEnumerable<string> -> unit + 1 overload
static member AppendAllText : path:string * contents:string -> unit + 1 overload
static member AppendText : path:string -> StreamWriter
static member Copy : sourceFileName:string * destFileName:string -> unit + 1 overload
static member Create : path:string -> FileStream + 3 overloads
static member CreateText : path:string -> StreamWriter
static member Decrypt : path:string -> unit
static member Delete : path:string -> unit
static member Encrypt : path:string -> unit
static member Exists : path:string -> bool
...
Full name: System.IO.File
System.IO.File.ReadAllBytes(path: string) : byte []
static member Util.Image : fileName:string -> BinaryOutput
static member Util.Image : bytes:seq<byte> * ?contentType:string -> BinaryOutput
static member Util.Image : bytes:seq<byte> * ?contentType:string -> BinaryOutput