The Display global
The Display
function is a 'global' function that can be accessed anywhere throughout
the notebook. It will take any object and attempt to display it to the user. Built-in
supported types are:
FSharp.Charting.ChartTypes.GenericChart
IfSharp.Kernel.GenericChartWithSize
IfSharp.Kernel.TableOutput
IfSharp.Kernel.HtmlOutput
IfSharp.Kernel.BinaryOutput
FSharp.Charting.ChartTypes.GenericChart
1: 2: |
let fruitData = [| ("Cherries", 100); ("Apples", 200); ("Bananas", 150); ("Peaches", 10) |] Chart.Bar(fruitData) |> Display |
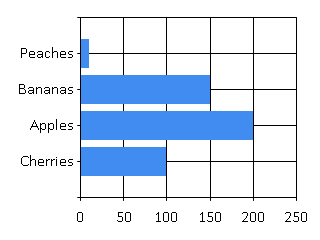
IfSharp.Kernel.GenericChartWithSize
1: 2: |
let moreFruitData = [| ("Cherries", 100); ("Apples", 200); ("Bananas", 150); ("Peaches", 10) |] Chart.Bar(moreFruitData) |> Chart.WithSize(640, 480) |> Display |
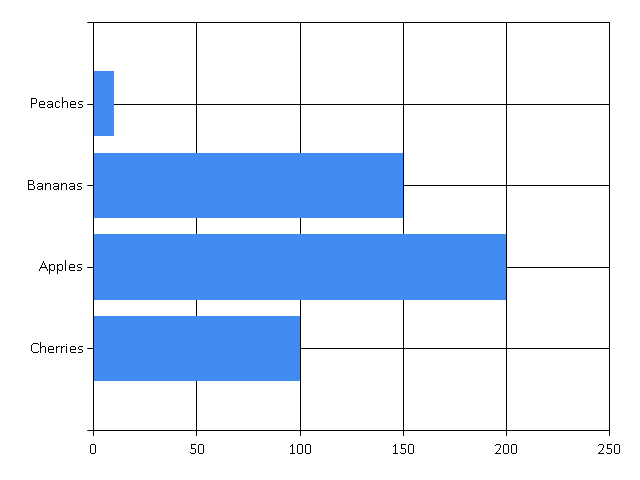
IfSharp.Kernel.TableOutput
This type is used for displaying data in an HTML table to the screen.
1: 2: 3: 4: 5: 6: 7: 8: 9: 10: 11: 12: 13: |
{ Columns = [| "First Name"; "Last Name" |] Rows = [| [| "Duane"; "Dibbley" |] [| "Arnold"; "Rimmer" |] [| "David"; "Lister" |] [| "Dave"; "Lister" |] [| ""; "Kryten" |] [| ""; "Holly" |] [| "Kristine"; "Kochanski" |] |] } |> Display |
First Name | Last Name |
---|---|
Duane | Dibbley |
Arnold | Rimmer |
David | Lister |
Dave | Lister |
Kryten | |
Holly | |
Kristine | Kochanski |
IfSharp.Kernel.HtmlOutput
This type is used for simply displaying a piece of HTML to the screen.
1: 2: 3: |
{ Html = @"<div style=""background-color: pink; font-weight: bold;"">Would you like some toast?</div>" } |> Display |
Would you like some toast?
IfSharp.Kernel.BinaryOutput
Used for displaying raw binary data with a content-type. All types are converted to this type when passed
through the Display
function. Supported content-types can be found on the ipython notebook documentation.
1: 2: 3: 4: |
{ ContentType = "image/png"; Data = System.IO.File.ReadAllBytes("img/toaster.png") } |> Display |
val fruitData : (string * int) []
Full name: Globals.fruitData
Full name: Globals.fruitData
type Chart = Chart
Full name: IfSharp.Kernel.Globals.Globals.Chart
Full name: IfSharp.Kernel.Globals.Globals.Chart
static member Chart.Bar : data:seq<#value> * ?Name:string * ?Title:string * ?Labels:#seq<string> * ?Color:System.Drawing.Color * ?XTitle:string * ?YTitle:string -> ChartTypes.GenericChart
static member Chart.Bar : data:seq<#key * #value> * ?Name:string * ?Title:string * ?Labels:#seq<string> * ?Color:System.Drawing.Color * ?XTitle:string * ?YTitle:string -> ChartTypes.GenericChart
static member Chart.Bar : data:seq<#key * #value> * ?Name:string * ?Title:string * ?Labels:#seq<string> * ?Color:System.Drawing.Color * ?XTitle:string * ?YTitle:string -> ChartTypes.GenericChart
val Display : ('a -> unit)
Full name: IfSharp.Kernel.Globals.Globals.Display
Full name: IfSharp.Kernel.Globals.Globals.Display
val moreFruitData : (string * int) []
Full name: Globals.moreFruitData
Full name: Globals.moreFruitData
static member Chart.WithSize : x:int * y:int -> (#ChartTypes.GenericChart -> GenericChartWithSize)
namespace Microsoft.FSharp.Data
namespace System
namespace System.IO
type File =
static member AppendAllLines : path:string * contents:IEnumerable<string> -> unit + 1 overload
static member AppendAllText : path:string * contents:string -> unit + 1 overload
static member AppendText : path:string -> StreamWriter
static member Copy : sourceFileName:string * destFileName:string -> unit + 1 overload
static member Create : path:string -> FileStream + 3 overloads
static member CreateText : path:string -> StreamWriter
static member Decrypt : path:string -> unit
static member Delete : path:string -> unit
static member Encrypt : path:string -> unit
static member Exists : path:string -> bool
...
Full name: System.IO.File
static member AppendAllLines : path:string * contents:IEnumerable<string> -> unit + 1 overload
static member AppendAllText : path:string * contents:string -> unit + 1 overload
static member AppendText : path:string -> StreamWriter
static member Copy : sourceFileName:string * destFileName:string -> unit + 1 overload
static member Create : path:string -> FileStream + 3 overloads
static member CreateText : path:string -> StreamWriter
static member Decrypt : path:string -> unit
static member Delete : path:string -> unit
static member Encrypt : path:string -> unit
static member Exists : path:string -> bool
...
Full name: System.IO.File
System.IO.File.ReadAllBytes(path: string) : byte []